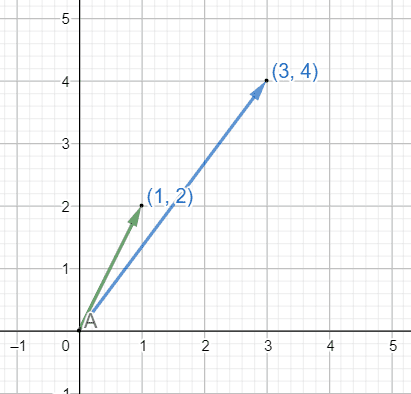
Math for Game Development and WebGL Part 2: Vector Multiplication
In the last post we talked about what a vector was, how to add and subtract vectors, and how to find the magnitude of a vector. We also demonstrated that by adding vectors we could translate (aka, move) a square around in two dimensions. Now we’ll look at scaling vectors using multiplication.
Multiply Vector by Scalar
The simplest vector multiplication is by a scalar value. As you could imagine from the name, a scalar value scales the vector. Remember a scalar value is just a regular number:
(3, 4) * 2 = (6, 8)
This is pretty straightforward, just multiply each component of the vector by the value. This vector is now double the length of the old vector, but is moving in the same direction
You can prove the length is the doubled with the Pythagorean theorem.
6² + 8² = c² 36 + 64 = c² √100 = c c = 10
This probably just makes intuitive sense, but we can verify that the magnitude (aka length aka size) of the vector has also doubled, not just the x and y components.
We could also shrink the vector by multiplying by a fraction. If we multiply (3, 4) by 0.5, we’ll get this:
Our vector is now half the length. We can also flip our vector by multiplying by a negative number:
Notice that by multiplying by a negative we can switch the direction!
Component Multiplication
We can also multiply each component of each vector by the matching component in the other. This will let us scale the x component at a different rate from the y component.
(3, 4) * (2, 1) = (6, 4)
We treat this just like addition or subtraction, they x component from both are multiplied together and the y component from both are multiplied together. Now we can scale in x and y, so lets add these to our Vector2 class and implement scaling in our square rendering. In the code below, I scale the square with the vector (2, 3), so the square should be twice as wide and three times as high. However, there’s a problem! Our square is gone!
See the Pen
Vector2 Class with Magnitude Method by Rob Louie (@rlouie)
on CodePen.
Can you tell why our square is gone? We’ve already translated our square to (100, 200), giving the squares upper left coordinate the position (100, 200). If we multiply (100, 200) by (2, 3), we get (200, 600). Well, our canvas is only 300 pixels tall. Our y position is now below the canvas. This leads us to an important discovery, scaling will scale the amount you’ve translated by if you’ve already translated. So the order here could matter, if we scaled first, we could then translate after and not get this effect. This is a little different from scale on the canvas, because scaling the canvas scales the entire grid system of the canvas, so order doesn’t really matter there. We’ll revisit that behavior shortly, and for now we will simply shorten the amount we translate by to keep the square on the screen.
See the Pen
Vector2 Class with Scaling Offscreen by Rob Louie (@rlouie)
on CodePen.
Our square isn’t a square anymore. Since we scaled it to double the width but triple the height, it’s become a rectangle. We have now both translated and scaled a shape on the canvas just using vector math! We could of course use the canvas scale and translate method, but with our knowledge of vector math we don’t have to. There’s still another transformation we need though, rotate. Unfortunately…that’s going to take some explaining…