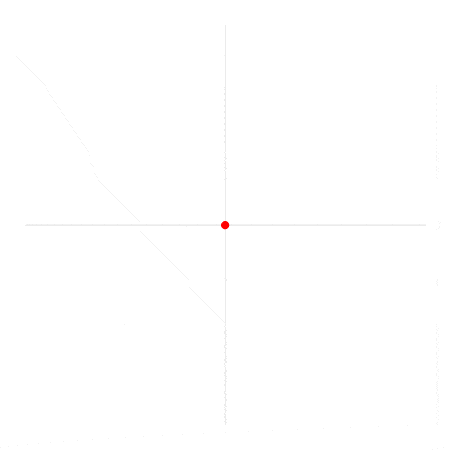
Math for Game Development and WebGL Part 3: Radians
We’re getting into rotation now which means we are going to be doing a lot of math with angles. When doing math with angles, you generally won’t use degrees like you may be used to. In math you generally want to use radians.
A radian measures the angle as related to the radius of the circle. In degrees we are used to 360° being a full circle. Can you guess how many radians make a full circle? If you remember how to find the circumference of a circle, you might know the answer.
The formula for the circumference of a circle is 2πr, or 2 times pi times the radius of the circle. The larger a circles radius, the larger the circumference, but no matter how large or small the circle is, it will still take 2π radii (radiuses) to go around the circle. That means it always takes 2π radians to go around a circle. This means that 360° is 2π radians. 180° is π radians, and so on. This can be seen in the animation below:
The idea that 360° is a full circle is a completely made up concept, it has no actual relation to a circle, other than the one we invented. Radians though are more mathematically “pure”, in that they are derived from the actual circle itself.
Degrees / Radians Conversion
While it’s definitely a good idea to start thinking in radians, that may take some getting used to, so it’s useful to be able to convert between the two. Luckily this is fairly easy.
We know that 2π radians === 360°, and can immediately simplify to π radians === 180°.
So one radian is 180/π degrees. I simply divide both sides by π to solve for 1 radian.
We can convert in the other direction by dividing both sides by 180 to solve for degrees.
So one degree is π/180 radians.
function degreesToRadians(degrees) { return degrees * (Math.PI / 180); } function radiansToDegrees(radians) { return radians * (180 / Math.PI); }
Introduction to the Unit Circle
While talking about some foundational angle/circle math, it’s a good time to at least introduce the unit circle. While this might sound super technical, it’s just a circle with a radius of one. We’ll see this being used more when we start doing more angle math, but I want to introduce it now. If a circle has a radius of one, what is it’s circumference? 2π! Or simply, the circumference is the same as the radians used to make a circle.
This can be very useful when doing math with angles and vectors because you can ignore the length of the vector and only focus on it’s direction. If you shorten the length of the vector to one, you can now find just it’s angle while ignoring it’s length. We’ll discuss this more later, but for now know that the unit circle is a circle with a radius of one, and that means it’s circumference is also 2π, the same as the number of radians to represent a full circle.
Summary
- A radian is a measurement of an angle that describes the angle relative to the radius of the circle
- The unit circle is a circle with the radius 1